One of the key aspects of building a React application is state management. When it comes to managing states in React, Redux and Context API are the two most common solutions. Although both are aimed at handling state updates, they have different features, use cases and setup processes. In this blog post you are going to learn what are the main differences between Redux vs Context API in ReactJS.
What is Redux?
“A JS library for predictable and maintainable global state management.”
Redux.js.org
Redux is a a state management container that provides a central “storage” to manage state in React applications. The key differentiation feature of Redux is that it ensures unidirectional data flow, which allows for high predictability, scalability and robustness. This makes it the perfect choice for developing large scale applications.
Key features of Redux
- Uniformity. The state logic of your entire React application is stored within a single object in the Redux store, which is the only source of state-related data.
- Immutability. With Redux, there is a defined logic according to which states are updated across the application. Changes are communicated through dispatching actions.
- Predictability. Redux manages states updates via reducers, which are functions that take the current state and return a new, updated state.
How to set up Redux
- Install Redux and React-Redux. To work with Redux in React, you will need both packages installed in your project.
npm install redux react-redux
JavaScript- Create a Redux store. In a new file, import createStore from Redux, then define an initial statue value, write the reducer function and create the store.
// store.js
import { createStore } from 'redux';
// Initial state
const initialState = {
count: 0
};
// Reducer
const counterReducer = (state = initialState, action) => {
if (action.type === 'increment') {
return { count: state.count + 1 };
} else if (action.type === 'decrement') {
return { count: state.count - 1 };
} else {
return state;
}
};
// Create store
const store = createStore(counterReducer);
export default store;
JavaScript- Create actions. Actions are responsible for sending data from the application components to the declared Redux store. You will need to declare actions meant to trigger new state updates.
// actions.js
export const increment = () => ({
type: 'increment'
});
export const decrement = () => ({
type: 'decrement'
});
JavaScript- Connect app to Redux. Import the Provider from react-redux to establish a connection between the React application and the Redux store. You will also need to import useSelector and useDispatch to interact with the state in the app.
// App.js
import React from 'react';
import { Provider, useSelector, useDispatch } from 'react-redux';
import store from './store';
import { increment, decrement } from './actions';
const Counter = () => {
const count = useSelector(state => state.count);
const dispatch = useDispatch();
return (
<div>
<p>{count}</p>
<button onClick={() => dispatch(increment())}>Increment</button>
<button onClick={() => dispatch(decrement())}>Decrement</button>
</div>
);
};
const App = () => (
<Provider store={store}>
<Counter />
</Provider>
);
export default App;
JavaScriptPros and Cons of Redux
Pros✅ | Cons❌ |
---|---|
Predictable state management due to unidirectional data flow. | Complexity overkilling small applications. |
Scalability for large-scale applications. | Learning curve for beginners. |
DevTools for debugging and monitoring state updates. | External libraries and imports. |
Middleware support for asynchronous action management. | Extensive boilerplate code. |
What is Context API?
“Context provides a way to pass data through the component tree without having to pass props down manually at every level.”
React.js.org
The Context API is a React build-in feature that allows sharing, updating and consuming state (“context”) across the app components without the hassle of having to manually pass down props at every level. Thanks to its functioning, Context API prevents props drilling and simplifies the code structure. ContextAPI vs Redux is much simpler and is therefore more suitable for smaller applications or for managing states in selected parts of large scale apps.
Key features of Context API
- React native. The Context API is a React built-in feature that does not require to be installed in the app, as in the case of Redux libraries.
- Structure. In ReactJs, the Context API follows a precise Provider – Consumer pattern. The Context Provider provides the state values to the components, and the Consumer components can then access this state.
- Simplicity. Thanks to its simplicity, the Context API is perfect for managing states in smaller React applications in an easy way.
How to set up Context API
- Create Context. The first step is creating a context that stores the application state and provides it to the app components. For this, you will need to import from React createContext and useState to manage state.
// CounterContext.js
import React, { createContext, useState } from 'react';
const CounterContext = createContext();
const CounterProvider = ({ children }) => {
const [count, setCount] = useState(0);
const increment = () => setCount(prevCount => prevCount + 1);
const decrement = () => setCount(prevCount => prevCount - 1);
return (
<CounterContext.Provider value={{ count, increment, decrement }}>
{children}
</CounterContext.Provider>
);
};
export { CounterContext, CounterProvider };
JavaScript- Consume Context in components. In the file containing all app components, wrap the entire application with Provider through useContext to allow the app components to consume the context, and therefore the state. For this, you will need to import useContext from React, as well as the previously declared Context and Context function.
// App.js
import React, { useContext } from 'react';
import { CounterProvider, CounterContext } from './CounterContext';
const Counter = () => {
const { count, increment, decrement } = useContext(CounterContext);
return (
<div>
<p>{count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
const App = () => (
<CounterProvider>
<Counter />
</CounterProvider>
);
export default App;
JavaScriptPros and Cons of Context API
Pros✅ | Cons❌ |
---|---|
Simplicity of set up. | Low performance due to frequent re-renders. |
Built-in React feature. | Lack of fixed structure. |
Suitability for small projects. | Unsuitability for large-scale projects. |
Ease of use. | Lack of middleware |
Redux vs Context API – Key differences
- Complexity. Managing state through Redux is more complex and requires more setup code in comparison to Context API. While with Context API you just need to create a context and then provide it to your components via a Provider, Redux involves the creation of a store, reducers, actions and the connection of components to the store. This makes Redux less suitable for small projects.
- Scalability. Redux allows for expansion thanks to its unique features and unidirectional flow of data management. Therefore, it is more suitable for large projects. In comparison, React built-in Context API is simpler and therefore ideal for small applications.
- Performance. While Redux minimizes re-renders by triggering state updates only in those components that require it, Context API triggers re-renders in all the subscribed components, also if unnecessary. This makes Redux more efficient than Context API.
Should I use Redux or Context API in React?
The choice depends on the requirements of your project. Use Redux for large scale projects with a high degree of complexity that require predictability and debugging tools. Use the built-in Context API for small-size applications that require a simple state management logic, as it is easier to set up and understand.
Bottom Line – Redux vs Context API?
Redux and Context API are two powerful solutions to manage state in React applications. While Context API does not require the installation of additional libraries and is easier to set up, Redux is more predictable and more efficient. You should use Context API for small projects and reserve Redux for large scale applications.
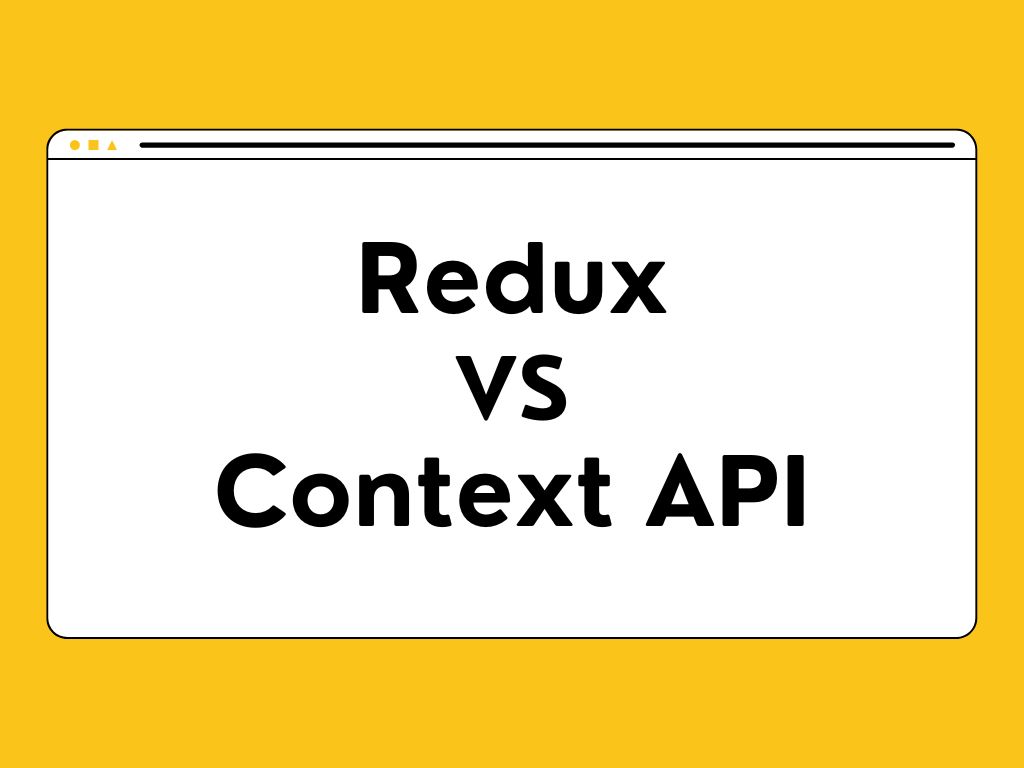